What is an API?
February 10, 2020
Overview
API is an abbreviation for Application Programming Interface. The key word is interface, and you're actually using one right now. Your phone or computer is a black box of software and hardware which you know very little (or nothing) about. Yet, you're controlling it within safe and secure limits through a user interface. APIs are no different in this respect; they're just designed for interaction between multiple black boxes of software.
Before we dive into examples, we need to split this discussion into the two most common types of black boxes of software where API is used to describe their points of connection:
- An application designed to handle communication over a network such as the internet: Web API.
- A library or framework that is intended to interact with another piece of code: Library or Framework API.
Other forms of APIs do exist, and we briefly mention them below in Other APIs.
Table of Contents
Examples
1. Web API
Say you're creating a chat app, and you want your app to import a user's Facebook photos. You'll need to use Facebook's Graph API to access their photos. Your app knows nothing about the software running on Facebook's servers, yet it's able to safely use their protected data and functionality thanks to Facebook's API.
An API is the collection of available connection points of a black box of software (note: single connection points can be referred to as APIs). It allows another black box of software to interact with the protected code and data behind the API. The two black boxes in the example were:
- a hypothetical chat app,
- and Facebook's server (their software exposed through their API).
This client-server architecture is one of the most prevalent design patterns in software:
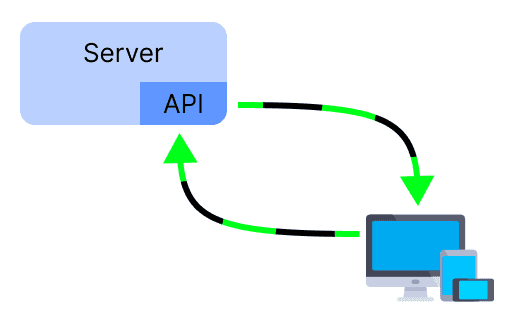
Note that a network is often simulated within the same machine using loopback connections.
The shape and structure of a Web API depends on its communication paradigm, for example:
- REST (architectural style that typically uses JSON)
- GraphQL (query language that models data as a tree and typically uses JSON)
- Protobuf (data serialization format)
REST | GraphQL | Protobuf | |
---|---|---|---|
Schema | No formal schema | Strongly typed schema | Strongly typed schema |
Request Structure | HTTP methods + URLs, JSON body | Single URL, GraphQL syntax text query | Varies, encoded to binary data |
Response Structure | JSON (typically) | JSON (matches query structure) | Varies, encoded to binary data |
APIs are often considered contracts for request and response structure between caller and callee.
REST
Example Request: Create Post (POST /posts)
{
"title": "New Post",
"content": "This is a post"
}
Example Response
{
"id": 1,
"title": "New Post",
"content": "This is a post"
}
GraphQL
Example Request
mutation {
createPost(title: "New Post", content: "This is a post") {
id
title
content
}
}
Example Response
{
"data": {
"createPost": {
"id": 1,
"title": "New Post",
"content": "This is a post"
}
}
}
Protobuf
Example Request
message CreatePostRequest {
string title = 1;
string content = 2;
}
Example Response
message CreatePostResponse {
Post post = 1;
}
message Post {
int32 id = 1;
string title = 2;
string content = 3;
}
2. Library or Framework API
Note: Frameworks are groups of libraries in addition to other tools (e.g. CLI programs) that help to provide a framework to build an application.
Library API
Black boxes of software don't have to physically be on different machines communicating over a network. Let's imagine you want to add a feature to your chat app where all numbers typed by users are converted to words, for example: 143 would become one hundred forty-three. You could implement this feature yourself, or you could find an open-source library that offers this functionality and include it in your app. This very basic library would provide a function that takes a number as an input and returns a string of characters as output. When that function executes, it executes code in the library. In this example, the library is the black box of software and the public function is its available connection point. That function is public because it's meant for you to import into your application by referencing it. This is in contrast to the library's private functions which are only meant to be used within the library. The collection of a library's public functions is its API.
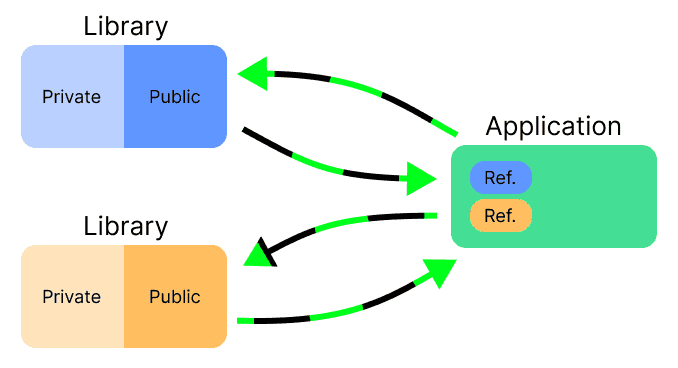
Once a library's public function is called, execution of the application's code moves to the library until a result (possibly none) is returned to the calling function. This is what importing a public function looks like in Python:
from path.to.code.my_module import my_function
# Calling my_function from my_module
result = my_function() # Could call it the result, response, or output (to name a few)
Framework API
Frameworks impose certain structures and conventions to help streamline development. The different ways that frameworks connect to the code in and around them (written by devs) constitute APIs. Their APIs typically fall into the following categories:
- Library APIs
- Directory Structure, File Naming, and/or Function Naming (Hook / Callback)
Learn more about Framework vs Library vs Package.
Directory Structure, File Naming, and/or Function Naming (Hook / Callback)
GatsbyJS has Gatsby Browser APIs where hooks can be defined that are invoked given various framework events. These hooks are invoked as callbacks within larger processes.
Other APIs
- Command Line Interfaces (CLIs) can be used by both humans and machines. In the latter case, they can be considered to operate as an API.
- SQL is a language that is designed to interact with SQL databases. The language itself can be considered an API (in a broad sense) for interacting with the database.
- Graphical User Interfaces (GUIs) are not typically considered APIs because they're intended to be used by humans, but when a scraper (for example) interacts with a GUI, this is a context where it could be considered an API in a broad sense of the term.
- Configuration Files are not typically considered APIs because they're not a point of contact between two or more components.
Conclusion
Software is a jungle of black boxes connected together by interfaces, but not all interfaces are APIs. The term API is not only dependent on context, it's also subjective. However, libraries and web applications are the most common black boxes of software whose points of connection are referred to as APIs.
This was a comprehensive, but not exhaustive, overview of what is an API.
Summary
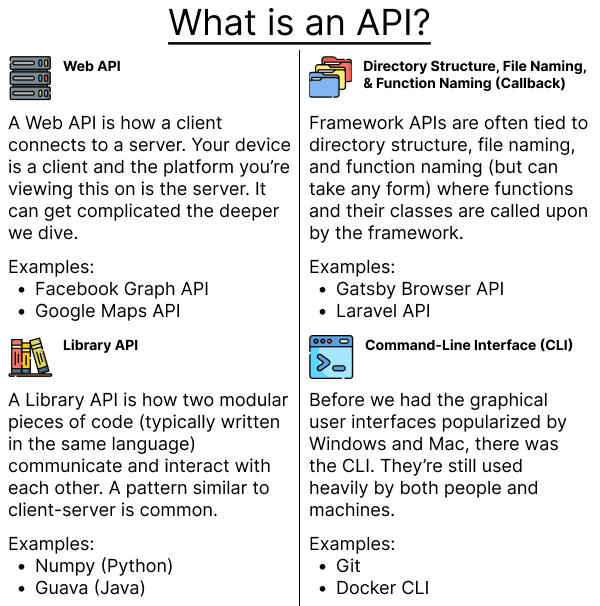
Related Posts
Updated: 2024-09-16